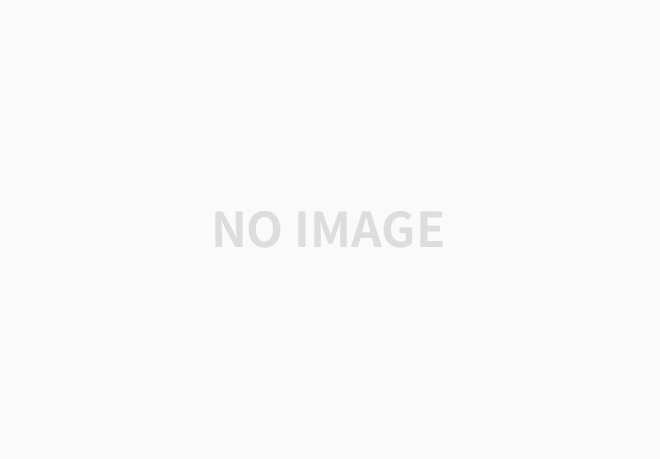
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
String title = scanner.nextLine();
float star = scanner.nextFloat();
scanner.nextLine(); //버퍼에 남아있는 \n을 가져와 비움
float percentage_star = (star / 5.0f) * 100;
String[] recipe = new String[10];
for (int i = 0; i < 10; i++){
recipe[i] = scanner.nextLine();
}
System.out.println(recipe[0]);
System.out.println("[ " + title + " ]");
System.out.println("별점 : " + (int)star + " (" + percentage_star + "%" + ")");
int index = 1;
for (int i = 0; i < 10; i++){
System.out.println( index++ + ". " + recipe[i]);
}
}
}
배열
향후 미리 저장할 값들을 선언하는 경우 : 타입[] 변수 = new 타입[길이];
문제점 및 해결
scanner.nextFloat(); 이후, 배열의 첫 번째 값이 입력되지 않음
- nextInt() 같은 메서드는 버퍼에 남아있는 연속된 숫자만 리턴하기 때문에, enter 값인 \n은 버퍼에 남겨둔다
- 그렇기 때문에 버퍼를 비워주는 작업을 통해서 해결함
float star = scanner.nextFloat();
scanner.nextLine(); //버퍼에 남아있는 \n을 가져와 비움
백분율을 구하는 부분에서 형변환 주의
- (star / 5.0f) 부분에서 나누는 수를 명시적으로 실수라고 지정해야 정확한 결과값이 나옴
'JAVA' 카테고리의 다른 글
Array / List / Stack / Queue / Set / Map (0) | 2025.01.02 |
---|---|
자바 기본 문법 / 얕은 복사와 깊은 복사 / String (1) | 2025.01.02 |
JAVA의 형변환과 자료형타입 (0) | 2025.01.01 |
JVM과 자바 컴파일 과정 (1) | 2024.12.31 |
보너스 문제: 가위 바위 보 (0) | 2024.12.17 |